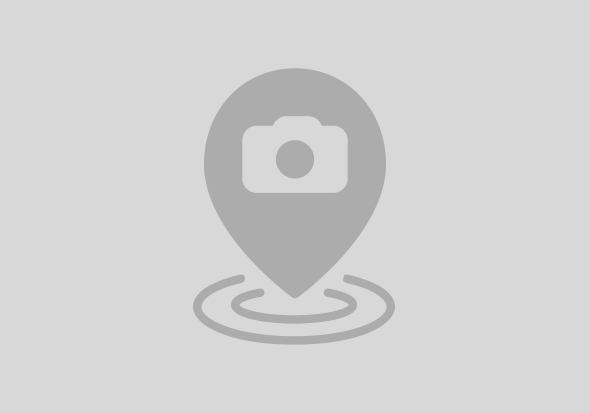
I saw recently a new post written by Roque that provide example on how to use Commit Params using Python API. So I decided to post the same thing in JAVA.
It’s similar to what Roque wrote but in Java.
Here the link to Maapi Doc
Here the link to CommitParams Doc
Basic Import that come with the generated package
import java.util.List;
import java.util.Properties;
import com.tailf.conf.*;
import com.tailf.navu.*;
import com.tailf.ncs.ns.Ncs;
import com.tailf.dp.*;
import com.tailf.dp.annotations.*;
import com.tailf.dp.proto.*;
import com.tailf.dp.services.*;
import com.tailf.ncs.template.Template;
import com.tailf.ncs.template.TemplateVariables;
Other import used for this example
//Logging
import org.apache.logging.log4j.LogManager;
import org.apache.logging.log4j.Logger;
//Socket for Action
import java.net.Socket;
// Maapi
import com.tailf.maapi.ApplyResult;
import com.tailf.maapi.CommitParams;
import com.tailf.maapi.DryRunResult;
import com.tailf.maapi.DryRunResult.DryRunEntry;
import com.tailf.maapi.Maapi;
import com.tailf.maapi.MaapiUserSessionFlag;
// Used in Action to get Host & PORT
import com.tailf.ncs.NcsMain;
I create a Logger object that will be used for logging
private static Logger LOGGER = LogManager.getLogger(testcommitparamservRFS.class);
Setting CommitParams:
General Steps:
Socket socket = new Socket(NcsMain.getInstance().getNcsHost(), NcsMain.getInstance().getNcsPort());
Maapi maapi = new Maapi(socket);
maapi.startUserSession("admin", maapi.getSocket().getInetAddress(), "system", new String[] { "admin" },
MaapiUserSessionFlag.PROTO_TCP);
int th = maapi.startTrans(Conf.DB_RUNNING, Conf.MODE_READ_WRITE);
2. Create params instance of CommitParams class:
In the example above , I will use cp to reference the CommitParams object.
CommitParams cp = maapi.getTransParams(th);
You can find here all the available method
3. Depending on the objective you format your params object. See examples below:
System.out.println(cp);
4) Apply and capture result:
applyTransParams(int tid, boolean keepOpen, CommitParams params) Apply a current transaction with transaction handle tid with additional NCS specific parameters. |
Link to ApplyTransParam doc
Example
ApplyResult result = maapi.applyTransParams(th, true, cp);
Example of possible parameters:
a) Commit dry-run outformat native use-lsa
cp.setDryRunNative();
cp.setUseLsa();
System.out.println(cp);
//Output:use-lsa trace-id=f52665a3-f461-41d6-8e56-3fc5e2787e14 dry-run/outformat=Enum(2)
Note: you can add as many as needed but some combinations would not make sense.
b) Commit reconcile:
cp.setReconcileDiscardNonServiceConfig();
//or
cp.setReconcileKeepNonServiceConfig();
c) Commit no-networking:
cp.setNoNetworking();
d) Commit no-deploy:
cp.setNoDeploy();
e) Set the trace-id:
cp.setTraceId("12345");
f) Commit no-out-of-sync-check:
cp.setNoOutOfSyncCheck();
g) Commit no-overwrite:
cp.setNoOverwrite();
h) Commit no-lsa:
cp.setNoLsa();
i) Commit dry-run outformat xml:
cp.setDryRunXml();
j) Commit dry-run outformat cli:
cp.setDryRunCli();
5) How to process dry-run result
Here link to java Doc of the class DryRunResult
Here link to java Doc of the class DryRunEntry
Make sure to import those class
import com.tailf.maapi.DryRunResult;
import com.tailf.maapi.DryRunResult.DryRunEntry;
import java.util.Iterator;
ApplyResult result = maapi.applyTransParams(th, true, cp);
if (result instanceof DryRunResult) {
DryRunResult dryrunResult = (DryRunResult) result;
Iterator<DryRunEntry> itr = dryrunResult.iterator();
int size = 0; // Variable to store how many element in dryrunResult
while (itr.hasNext()) {
DryRunEntry element = itr.next();
LOGGER.info("Name: {} | Type : {} | Data : {}", element.getName(),element.getTypeAsString(), + element.getData());
size++;
}
LOGGER.info ("dryrunResult Size: {}", size);
}
7) Close Transaction
maapi.finishTrans(th);// Finish the transaction
maapi.endUserSession(); // Close user session
socket.close();// Close the Socket
How to access to CommitParams from service Code in Java:
Check if it’s a dry-run and other commit parameters:
Note: Please remember to avoid side-effects as a well-known best practice.
Some examples based on the java-and service skeleton service:
I iterate through all available method in the CommitParams object to get all getter method that startwith “is” or “get” except the getClass since it’s part of Object class.
I define a set methodToSkip where I add to the method getClass in order to Skip from being called inside the loop.
//Logging
import org.apache.logging.log4j.LogManager;
import org.apache.logging.log4j.Logger;
import java.lang.reflect.Method;
import java.util.HashSet;
import java.util.Set;
@ServiceCallback(servicePoint = "test-commit-param-serv-servicepoint", callType = ServiceCBType.CREATE)
public Properties create(ServiceContext context, NavuNode service, NavuNode ncsRoot, Properties opaque)
throws ConfException {
Template myTemplate = new Template(context, "test-commit-param-serv-template");
TemplateVariables myVars = new TemplateVariables();
Set<String> methodToSkip = new HashSet<String>();
methodToSkip.add("getClass"); // Skip GetClass method that's is present in all Java Object
// https://docs.oracle.com/javase/8/docs/api/java/lang/Object.html
try {
NavuContext nContext = service.context();
Maapi m = nContext.getMaapi();
int th = nContext.getMaapiHandle();
CommitParams cp = m.getTransParams(th); // https://developer.cisco.com/docs/nso/api/#!com-tailf-maapi/package%c2%a0com-tailf-maapi
// https://pubhub.devnetcloud.com/media/nso-api-docs
/docs/5.8/java/com/tailf/maapi/CommitParams.html
System.out.println(cp);
LOGGER.info("CommitParams Object :{}", cp);
LOGGER.info("Check dry-run:" + cp.isDryRun());
if (cp.isDryRun()) {
LOGGER.info("Dry-run outformat Name:" + cp.getDryRunOutformat().name());
LOGGER.info("Dry-run outformat Value:" + cp.getDryRunOutformat());
// LOGGER.info("Dry-run outformat" +cp.getDryRunOutformat());
}
LOGGER.info("Get Trace-id:" + cp.getTraceId());
LOGGER.info("Check use-lsa:" + cp.isUseLsa());
LOGGER.info("Check no-networking:" + cp.isNoNetworking());
if (cp.isReconcileKeepNonServiceConfig() || cp.isReconcileDiscardNonServiceConfig()) {
LOGGER.info("Reconciliation is On");
} else {
LOGGER.info("Reconciliation is Off");
}
Class objClass = cp.getClass();
Method[] methods = objClass.getMethods();
for (Method method : methods) {
// if ((method.getName().startsWith("get") || method.getName().startsWith("is"))
if (method.getName().matches("(is|get).*") && !methodToSkip.contains(method.getName())) {
LOGGER.info("Calling method {} =====> got {}", method.getName(), method.invoke(cp));
}
}
} catch (Exception e) {
LOGGER.error("Exception during getting CommitParams", e);
}
try {
// set a variable to some value
myVars.putQuoted("DUMMY", "10.0.0.1");
// apply the template with the variable
myTemplate.apply(service, myVars);
} catch (Exception e) {
throw new DpCallbackException(e.getMessage(), e);
}
return opaque;
}
Here example of the log that I got
<INFO> 25-Apr-2022::20:48:16.498 testcommitparamservRFS Did-243-Worker-48: - CommitParams Object :use-lsa trace-id=f52665a3-f461-41d6-8e56-3fc5e2787e14 dry-run/outformat=Enum(2)
<INFO> 25-Apr-2022::20:48:16.499 testcommitparamservRFS Did-243-Worker-48: - Check dry-run:true
<INFO> 25-Apr-2022::20:48:16.499 testcommitparamservRFS Did-243-Worker-48: - Dry-run outformat Name:NATIVE
<INFO> 25-Apr-2022::20:48:16.499 testcommitparamservRFS Did-243-Worker-48: - Dry-run outformat Value:2
<INFO> 25-Apr-2022::20:48:16.499 testcommitparamservRFS Did-243-Worker-48: - Get Trace-id:f52665a3-f461-41d6-8e56-3fc5e2787e14
<INFO> 25-Apr-2022::20:48:16.499 testcommitparamservRFS Did-243-Worker-48: - Check use-lsa:true
<INFO> 25-Apr-2022::20:48:16.499 testcommitparamservRFS Did-243-Worker-48: - Check no-networking:false
<INFO> 25-Apr-2022::20:48:16.499 testcommitparamservRFS Did-243-Worker-48: - Reconciliation is Off
<INFO> 25-Apr-2022::20:48:16.499 testcommitparamservRFS Did-243-Worker-48: - Calling method isCommitQueueAsync =====> got false
<INFO> 25-Apr-2022::20:48:16.499 testcommitparamservRFS Did-243-Worker-48: - Calling method isCommitQueueSync =====> got false
<INFO> 25-Apr-2022::20:48:16.500 testcommitparamservRFS Did-243-Worker-48: - Calling method getCommitQueueSyncTimeout =====> got null
<INFO> 25-Apr-2022::20:48:16.500 testcommitparamservRFS Did-243-Worker-48: - Calling method isCommitQueueBypass =====> got false
<INFO> 25-Apr-2022::20:48:16.500 testcommitparamservRFS Did-243-Worker-48: - Calling method getCommitQueueTag =====> got null
<INFO> 25-Apr-2022::20:48:16.500 testcommitparamservRFS Did-243-Worker-48: - Calling method isCommitQueueLock =====> got false
<INFO> 25-Apr-2022::20:48:16.500 testcommitparamservRFS Did-243-Worker-48: - Calling method isCommitQueueBlockOthers =====> got false
<INFO> 25-Apr-2022::20:48:16.500 testcommitparamservRFS Did-243-Worker-48: - Calling method isCommitQueueAtomic =====> got false
<INFO> 25-Apr-2022::20:48:16.500 testcommitparamservRFS Did-243-Worker-48: - Calling method isCommitQueueNonAtomic =====> got false
<INFO> 25-Apr-2022::20:48:16.500 testcommitparamservRFS Did-243-Worker-48: - Calling method getCommitQueueErrorOption =====> got null
<INFO> 25-Apr-2022::20:48:16.500 testcommitparamservRFS Did-243-Worker-48: - Calling method isNoRevisionDrop =====> got false
<INFO> 25-Apr-2022::20:48:16.500 testcommitparamservRFS Did-243-Worker-48: - Calling method isNoOverwrite =====> got false
<INFO> 25-Apr-2022::20:48:16.500 testcommitparamservRFS Did-243-Worker-48: - Calling method isNoOutOfSyncCheck =====> got false
<INFO> 25-Apr-2022::20:48:16.501 testcommitparamservRFS Did-243-Worker-48: - Calling method isNoLsa =====> got false
<INFO> 25-Apr-2022::20:48:16.501 testcommitparamservRFS Did-243-Worker-48: - Calling method isNoDeploy =====> got false
<INFO> 25-Apr-2022::20:48:16.501 testcommitparamservRFS Did-243-Worker-48: - Calling method isDryRunReverse =====> got false
<INFO> 25-Apr-2022::20:48:16.501 testcommitparamservRFS Did-243-Worker-48: - Calling method getWaitDevice =====> got []
<INFO> 25-Apr-2022::20:48:16.501 testcommitparamservRFS Did-243-Worker-48: - Calling method isDryRun =====> got true
<INFO> 25-Apr-2022::20:48:16.502 testcommitparamservRFS Did-243-Worker-48: - Calling method getConfXMLParam =====> got [{[1145948134|use-lsa], LEAF}, {[1145948134|trace-id], f52665a3-f461-41d6-8e56-3fc5e2787e14}, {[1145948134|dry-run], START}, {[1145948134|outformat], Enum(2)}, {[1145948134|dry-run], STOP}, {[1145948134|reconcile], LEAF}]
<INFO> 25-Apr-2022::20:48:16.502 testcommitparamservRFS Did-243-Worker-48: - Calling method getDryRunOutformat =====> got 2
<INFO> 25-Apr-2022::20:48:16.502 testcommitparamservRFS Did-243-Worker-48: - Calling method getTraceId =====> got f52665a3-f461-41d6-8e56-3fc5e2787e14
<INFO> 25-Apr-2022::20:48:16.502 testcommitparamservRFS Did-243-Worker-48: - Calling method isUseLsa =====> got true
<INFO> 25-Apr-2022::20:48:16.502 testcommitparamservRFS Did-243-Worker-48: - Calling method isNoNetworking =====> got false
<INFO> 25-Apr-2022::20:48:16.502 testcommitparamservRFS Did-243-Worker-48: - Calling method isReconcileKeepNonServiceConfig =====> got false
<INFO> 25-Apr-2022::20:48:16.502 testcommitparamservRFS Did-243-Worker-48: - Calling method isReconcileDiscardNonServiceConfig =====> got false
You must be a registered user to add a comment. If you've already registered, sign in. Otherwise, register and sign in.
Find answers to your questions by entering keywords or phrases in the Search bar above. New here? Use these resources to familiarize yourself with the NSO Developer community: